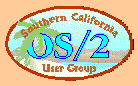 |
Next Meeting: Sat, TBD
Meeting Directions
|
Navigation:
20 Most Recent Documents
Search Archives
Index by date,
title,
author,
category.
|
|
Features:
Mr. Know-It-All
Ink
Download!
|
|
|
|
|
|
|
SCOUG:
Home
Email Lists
SIGs (Internet, General Interest, Programming, Network, more..)
Online Chats
Business
Past Presentations
Credits
Submissions
Contact SCOUG
Copyright SCOUG
|
|
Pictures from Sept. 1999
|
|
The views expressed in articles on this site are those of their authors.
|
SCOUG was there!
|
|
Copyright 1998-2025, Southern California OS/2 User Group.
ALL RIGHTS RESERVED.
SCOUG, Warp Expo West, and Warpfest are trademarks of the
Southern California OS/2 User Group.
OS/2, Workplace Shell, and IBM are registered trademarks of
International Business Machines Corporation.
All other trademarks remain the property of their respective owners.
The Southern California OS/2 User Group
USA
|
|
|
Using REXX and C Together - Part 1
Stan Shantar, Orange Hill Software and SCOUG Member
|
- Introduction
- The OS/2 Warp REXX Implementation
- Using REXX to Supplement a C Application
- Using C to Provide Enhanced Capabilities for REXX programs
- How REXX Is Implemented In OS/2 Warp
- Reentrant interpreter - REXX.DLL and REXXINIT.DLL
- The REXX-C interface - REXXAPI.DLL
- Advanced REXX functions - REXXUTIL.DLL
- Simple development tools - PMREXX.EXE and REXXTRY.CMD
- Using REXX To Supplement C - part 1
- Allow users to add custom processing routines (scripts)
- Provide overrideable execution options for field support
- For non-PM programs, use REXX's character-based UI functions
- Use REXX where REXX is better/easier than C!
- Using C For Enhancing REXX - part 2
- Provide additional functions to REXX programs
- Create Database, Communication, and Network interfaces
- Provide PM GUI functionality
- Provide advanced OS features (threads, semaphores, IPC)
- Online Reference Materials
- OS/2 Prodcedures Language 2/REXX (comes with Warp)
- REXX Program Reference or Object REXX Programming Guide
(comes with Developer's Toolkit and Visual Age C++)
- Procedures Language 2/REXX User's Guide (REXXBASE.INF)
- The RXSTRING Structure
- Definition:
typedef struct {
ULONG strlength; /* length of string */
PCH strptr; /* pointer to string */
} RXSTRING;
typedef RXSTRING *PRXSTRING; /* pointer to a RXSTRING */
- Starting REXX From a C Application
- The RexxStart function prototype:
LONG APIENTRY RexxStart( LONG, /* Arg count (argc) */
PRXSTRING, /* Array of args (argv) */
PSZ, /* REXX program name */
PRXSTRING, /* INSTORE array */
PSZ, /* Initial environment */
LONG, /* type (cmd/sub/funct) */
PRXSYSEXIT, /* SysExit array */
PSHORT, /* Return code */
PRXSTRING ); /* Returned value */
- Preparing To Call RexxStart
- Build RXSTRINGS for arguments to be passed
- Build RXSTRING for returned value
- Thread calling RexxStart waits for REXX program to end
- A Simple RexxStart Example
RXSTRING rxsArgs[2];
RXSTRING rxsReturn;
SHORT sReturn;
CHAR szArg1[4], szArg2[4];
strcpy(szArg1, "A");
rxsArgs[0].strptr = szArg1;
rxsArgs[0].strlength = strlen(szArg1);
strcpy(szArg2, "B");
rxsArgs[1].strptr = szArg2;
rxsArgs[1].strlength = strlen(szArg2);
rxsReturn.strptr = szReturn;
rxsReturn.strlength = sizeof(szReturn);
rc = RexxStart(2, rsxArgs, "sample.cmd", NULL,
"CMD", RXFUNCTION, NULL,
&sReturn, &rxsReturn);
- Return Codes and Values
- RC from RexxStart function
- RC from REXX procedure (if numeric)
- Returned value from REXX procedure
- Automatic storage allocation for return
- MUST check if REXX allocated any storage
- INSTORE Options
- NULL array
- REXX program to be loaded from disk
- INSTORE[0]
- RXSTRING describing REXX source
- INSTORE[1]
- RXSTRING describing tokenized image
- If INSTORE[1] is empty, tokenized image will be returned
- If BOTH are empty, REXX will search the macrospace
- Preloading for Enhanced Performance
- Single argument of "//T" will tokenize without executing
- Tokenized image is returned in INSTORE[1]
- Subsequent calls to RexxStart will run tokenized image
- Acquired storage MUST be freed when no longer needed
- Embedding REXX Source As A Resource
- Reference actual REXX source file in .rc file
- DosLoadResource and DosQueryResourceSize to build INSTORE[0]
- Call RexxStart with "//T" argument and empty INSTORE[1]
- For ultimate performance, use a thread during startup
- System Exits
- A function that is to be called during REXX execution
- Exit types are used to specify WHEN exit is called
- Exits must be registered before used
- May be either within the executable or in a DLL
- Exits in effect are declared in each RexxStart invocation
- System Exit Types
- RXFNC - RXFNCCAL
- RXCMD - RXCMDHST
- RXMSQ - RXMSQPLL
RXMSQPSH
RXMSQSIZ
RXMSQNAM
- RXSIO - RXSIOSAY
RXSIOTRC
RXSIOTRD
RXSIODTR
- RXHLT - RXHLTTST
RXHLTCLR
- RXTRC - RXTRCTST
- RXINI - RXINIEXT
- RXTER - RXTEREXT
- System Exit Functions
- ALL exit functions MUST be defined as follows:
LONG EXPENTRY MyExitHandler( LONG lExit, /* exit type */
LONG lSubfunction, /* exit subfunction */
PEXIT pParmBlock ); /* parameters */
- Register with RexxRegisterExitExe or RexxRegisterExitDll
- RXSYSEXIT structure (used in RexxStart):
typedef struct _RXSYSEXIT {
PSZ sysexit_name; /* registered name of handler */
LONG sysexit_code; /* exit type */
} RXSYSEXIT;
- The Variable Pool Interface
- REXX variables are stored as name-value string pairs
- Variable Pool interface allows C program to set and fetch values
- Fetch either by explicit name or enumeration
- Multiple requests can be made in a single call to RexxVariablePool
- Two types of name specification: Symbolic and Direct
- The SHVBLOCK Structure
- Used to create a linked list of requests
- Definition:
typedef struct _SHVBLOCK {
struct _SHVBLOCK *shvnext; /* pointer to the next block */
RXSTRING shvname; /* name buffer */
RXSTRING shvvalue; /* value buffer */
ULONG shvnamelen; /* length of the name */
ULONG shvvaluelen; /* length of the value */
UCHAR shvcode; /* operation code */
UCHAR shvret; /* return code flags s*/
} SHVBLOCK;
typedef SHVBLOCK *PSHVBLOCK;
- Operation Codes and Return Flags
- Operation codes:
- RXSHV_SYSET
- set
- RXSHV_SYFETCH
- fetch
- RXSHV_SYDRO
- drop
- RXSHV_NEXTV
- enumerate variables
- RXSHV_PRIV
- fetch private information
- Return flags:
- RXSHV_OK
- RXSHV_NEWV
- RXSHV_LVAR
- RXSHV_TRUNC
- The RexxVariablePool Function
- Function prototype:
APIRET APIENTRY RexxVariablePool(PSHVBLOCK);
- Single argument - Pointer to 1st SHVBLOCK in list
- Return code is aggregate of all return flags in list
- Use of RexxVariablePool in System Exits
- Initialize variables in a RXINI system exit function
- Retrieve variable values in a RXTER system exit function
- Multiple thread considerations
|
|
|